对我来说,并不需要什么特别复杂的功能,简单实现一下以下功能就可以了。
- 主机信息显示(显示当前设备剩余内存、CPU占用、磁盘占用等等信息)
- 设备信息卡(设备是否在线,控制设备,如LED,风扇等等控制内容)
- 任务日程显示(显示设备的计划任务日期)
- 设置页面(设置系统信息之类的)
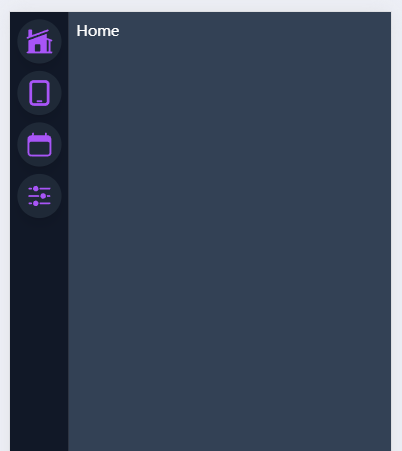
大概是这样的一个界面效果。这样的效果其实并不难实现,可以使用Vue
+ Tailwind css
+ Heroicons
就可以轻松实现了。
首先我们用pnpm 创建一个vite的模板工程。
创建好的工程目录大致如下(我添加了views目录)
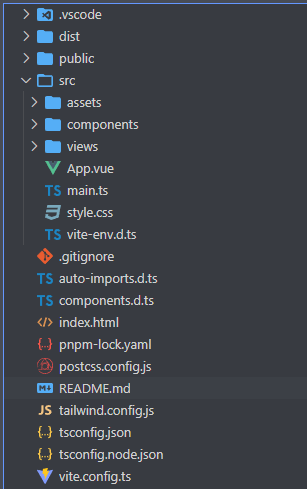
然后我们需要修改main.ts文件,并将路由设置好
import { createRouter, createWebHistory } from 'vue-router'
const router = createRouter({
history: createWebHistory(),
routes: [
{ path: '/', name: 'home', component: () => import('./views/home.vue') },
{ path: '/devices', name: 'devices', component: () => import('./views/devices.vue') },
{ path: '/calendar', name: 'calendar', component: () => import('./views/calendar.vue') },
{ path: '/config', name: 'config', component: () => import('./views/config.vue') },
]
})
createApp(App).use(router).mount('#app')
然后在views目录下新建对应的home.vue等等文件,暂时先用简单的模板来填充。
<template>
<h1 class="m-1 p-1">Home</h1>
</template>
接下来,我们需要制作导航栏,导航栏的内容如下
<template>
<div id="nav">
<RouterLink to="/" class="sidebar-icon">
<HomeModernIcon class="w-8 h-8" />
<span class="sidebar-tooltip group-hover:scale-100">主页</span>
</RouterLink>
<RouterLink to="/devices" class="sidebar-icon">
<DeviceTabletIcon class="w-8 h-8" />
<span class="sidebar-tooltip group-hover:scale-100">设备列表</span>
</RouterLink>
<RouterLink to="/calendar" class="sidebar-icon">
<CalendarIcon class="w-8 h-8" />
<span class="sidebar-tooltip group-hover:scale-100">任务日程</span>
</RouterLink>
<RouterLink to="/config" class="sidebar-icon">
<AdjustmentsHorizontalIcon class="w-8 h-8" />
<span class="sidebar-tooltip group-hover:scale-100">系统设置</span>
</RouterLink>
</div>
</template>
<script lang="ts" setup>
import { HomeModernIcon, DeviceTabletIcon, CalendarIcon, AdjustmentsHorizontalIcon } from '@heroicons/vue/24/solid'
</script>
在这里使用了Heroicons
内置的图标,这是一个可以免费使用的开源图标库,内置了280多个图标,我们可以用它来美化页面。
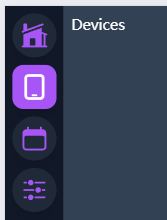
默认是圆型图标,点击后图标范围扩大成圆角方形图标,有简单的动态效果,实现这些效果的是以下的css配置文件。
@layer components {
.sidebar-icon {
@apply relative flex items-center justify-center
h-12 w-12 mt-2 mb-2 mx-auto shadow-lg
bg-gray-800 text-purple-500
hover:bg-purple-500 hover:text-white
rounded-3xl hover:rounded-xl
transition-all duration-150 ease-linear
cursor-pointer;
}
.sidebar-tooltip {
@apply absolute w-auto p-3 m-3 min-w-max left-14
rounded-md shadow-md
text-white bg-gray-900
text-xs font-bold
transition-all duration-100 scale-0 origin-left;
}
}
这个代码是使用了Tailwind css的现成样式,习惯了Tailwind css,它的样式名称和功能是对应的。可以帮助我们快速构建界面而无需编写自定义CSS。
app.vue文件的内容也比较简单,仅仅是将页面拆分成导航栏和内容栏两个框而已。
<script setup lang="ts">
import Nav from './views/nav.vue'
</script>
<template>
<div class="fixed top-0 left-0 h-screen w-16 m-0
flex flex-col
bg-gray-900 text-white shadow-lg">
<Nav />
</div>
<div class="fixed top-0 left-16 h-screen w-full m-0 bg-slate-700 text-white">
<RouterView />
</div>
</template>
这样一个简单的页面,我一边学习一边写,也就花了半个小时左右。构建出来的页面体积也很小,压缩后不到40KB。
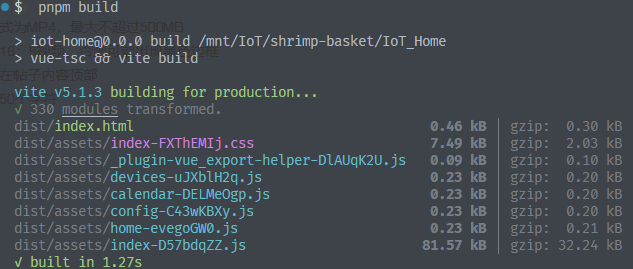
明天我会继续编写主机信息显示卡的内容,用来显示当前Linux主机的一些状态信息。