VE文件引脚定义
ct_trigger和full_sign两个信号线
首先是act_trigger和full_sign为两个信号线,我们分别将其映射到GPIO2_1和GPIO2_2上。其中,act_trigger方向为MCU->CPLD;full_sign方向为CPLD->MCU。因此其定义如下:
GPIO2_1 act_trigger:OUTPUT
GPIO2_2 full_sign:INPUT
- 当控制mcu 的gpio2_1 高低切换时,cpld 中的act_trigger_out_data,会对应来变化。
- 当cpld 中控制full_sign_in_data 信号高低时,mcu 中的gpio2_2 对应变化。
8个采样引脚
根据板卡上的排针排列,我们选取的采样引脚对应关系如下:
data_in[7] |
data_in[6] |
data_in[5] |
data_in[4] |
data_in[3] |
data_in[2] |
data_in[1] |
data_in[0] |
---|
PIN95 |
PIN92 |
PIN88 |
PIN86 |
PIN84 |
PIN82 |
PIN80 |
PIN67 |
VE文件编写如下:
data_in[0] PIN_67:INPUT
data_in[1] PIN_80:INPUT
data_in[2] PIN_82:INPUT
data_in[3] PIN_84:INPUT
data_in[4] PIN_86:INPUT
data_in[5] PIN_88:INPUT
data_in[6] PIN_92:INPUT
data_in[7] PIN_95:INPUT
MCU代码编写
if(buf[0] == 0x01)//写入设置
{
int wValue = 0;
// 将 buf[0] 放入 int 的低8位
wValue |= buf[1];
// 将 buf[1] 移动到 int 的中间8位,并与combined进行或运算
wValue |= (buf[2] << 8);
//write register 0x60000008
*((int *)0x60000008) = wValue;
}
else if(buf[0] == 0x02)
{
//GPIO_SetLow(SIGNAL_GPIO, ACT_GPIO_BITS);
//GPIO_SetHigh(LED_GPIO, ACT_GPIO_BITS);
for(int i = 0; i < 4096; i++)
{
buf[i] = *((char *)0x60000004);
asm("nop");
}
cdc_transfer(buf, 4096);
}
act_en: MCU->CPLD 当进行读取FIFO时将其拉低
full:CPLD->MCU FIFO满标志位,当full=1时,将act_en拉低,并且读出FIFO中全部数据
上位机代码
import serial
import struct
import numpy as np
import matplotlib.pyplot as plt
class LogicAnalyzer:
def __init__(self, channels):
self.channels = channels
self.data = {channel: [] for channel in channels}
def capture(self, signals):
for channel, signal in zip(self.channels, signals):
self.data[channel].append(signal)
def plot(self):
time = np.arange(0, len(self.data[self.channels[0]]))
for index, channel in enumerate(self.channels):
plt.subplot(len(self.channels), 1, index+1)
plt.step(time, self.data[channel], where='post', label=channel)
plt.xlabel('Time')
plt.ylabel('Signal Level')
plt.title('Logic Analyzer Output')
plt.legend()
plt.grid()
plt.show()
ser = serial.Serial(
port='COM17',
baudrate=9600,
parity=serial.PARITY_NONE,
stopbits=serial.STOPBITS_ONE,
bytesize=serial.EIGHTBITS,
timeout=2
)
try:
number_to_send = 2
packed_number = struct.pack('>B', number_to_send)
ser.write(packed_number)
total_received = b''
expected_size = 4096
while len(total_received) < expected_size:
remaining = expected_size - len(total_received)
chunk = ser.read(min(remaining, 1024))
if not chunk:
break
total_received += chunk
if len(total_received) == expected_size:
print("Received all data.")
bits0 = [byte & 1 for byte in total_received]
bits1 = [(byte >> 1) & 1 for byte in total_received]
bits2 = [(byte >> 2) & 1 for byte in total_received]
bits3 = [(byte >> 3) & 1 for byte in total_received]
bits4 = [(byte >> 4) & 1 for byte in total_received]
bits5 = [(byte >> 5) & 1 for byte in total_received]
bits6 = [(byte >> 6) & 1 for byte in total_received]
bits7 = [(byte >> 7) & 1 for byte in total_received]
print("Extracted lowest bits:")
else:
print("Did not receive enough data. Received:", len(total_received), "bytes out of", expected_size, "expected.")
except Exception as e:
print(f"Error occurred: {e}")
finally:
ser.close()
analyzer = LogicAnalyzer(['Channel 1', 'Channel 2', 'Channel 3', 'Channel 4', 'Channel 5', 'Channel 6', 'Channel 7', 'Channel 8'])
for i in range(4096):
signals = [bits0[i], bits1[i], bits2[i], bits3[i], bits4[i], bits5[i], bits6[i], bits7[i]]
analyzer.capture(signals)
analyzer.plot()
结果
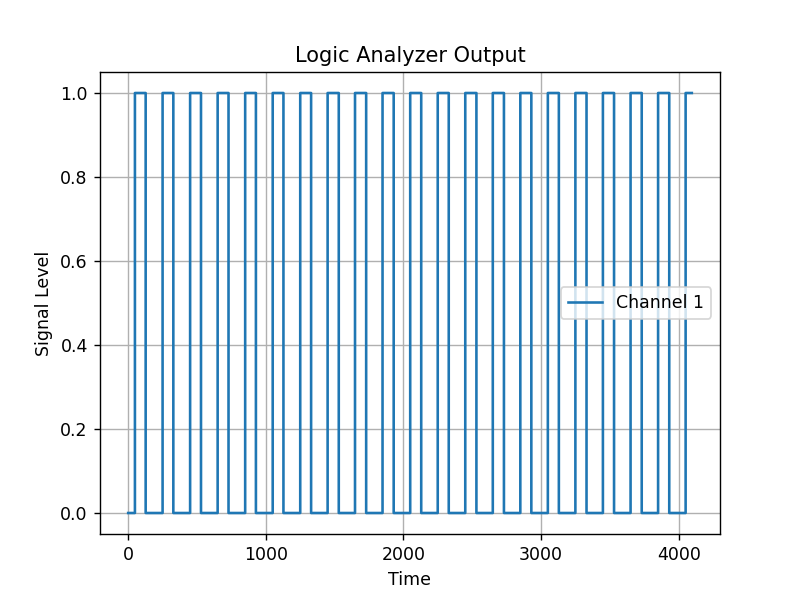