C语言交叉编译控制LED
参考教程:https://spotpear.cn/index/study/detail/id/1137.html
1、进入milkv,cat /proc/version

官方使用riscv64-unknown-linux-musl-gcc编译器,个人编译时也需要使用相同编译器。
2、为方便管理,在SDK下创建test文件夹,编写led.c
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#define SYSFS_GPIO_EXPORT "/sys/class/gpio/export"
#define SYSFS_GPIO_UNEXPORT "/sys/class/gpio/unexport"
#define SYSFS_GPIO_RST_PIN_VAL "440"
#define SYSFS_GPIO_RST_DIR "/sys/class/gpio/gpio440/direction"
#define SYSFS_GPIO_RST_DIR_VAL "OUT"
#define SYSFS_GPIO_RST_VAL "/sys/class/gpio/gpio440/value"
#define SYSFS_GPIO_RST_VAL_H "1"
#define SYSFS_GPIO_RST_VAL_L "0"
int main()
{
int fd;
int count = 30;
fd = open(SYSFS_GPIO_EXPORT, O_WRONLY);
if (fd == -1)
{
printf("ERR: export open error.\n");
return EXIT_FAILURE;
}
write(fd, SYSFS_GPIO_RST_PIN_VAL, sizeof(SYSFS_GPIO_RST_PIN_VAL));
close(fd);
fd = open(SYSFS_GPIO_RST_DIR, O_WRONLY);
if (fd == -1)
{
printf("ERR: direction open error.\n");
return EXIT_FAILURE;
}
write(fd, SYSFS_GPIO_RST_DIR_VAL, sizeof(SYSFS_GPIO_RST_DIR_VAL));
close(fd);
fd = open(SYSFS_GPIO_RST_VAL, O_RDWR);
if (fd == -1)
{
printf("ERR: gpio open error.\n");
return EXIT_FAILURE;
}
while (count)
{
count--;
printf("milk:%d\r\n",count);
write(fd, SYSFS_GPIO_RST_VAL_H, sizeof(SYSFS_GPIO_RST_VAL_H));
usleep(1000000);
write(fd, SYSFS_GPIO_RST_VAL_L, sizeof(SYSFS_GPIO_RST_VAL_L));
usleep(1000000);
}
close(fd);
fd = open(SYSFS_GPIO_UNEXPORT, O_WRONLY);
if (fd == -1)
{
printf("ERR: unexport open error.\n");
return EXIT_FAILURE;
}
write(fd, SYSFS_GPIO_RST_PIN_VAL, sizeof(SYSFS_GPIO_RST_PIN_VAL));
close(fd);
return 0;
}
3、在电脑ubuntu查找对应的编译器
sudo find / | grep riscv64-unknown-linux-musl-gcc
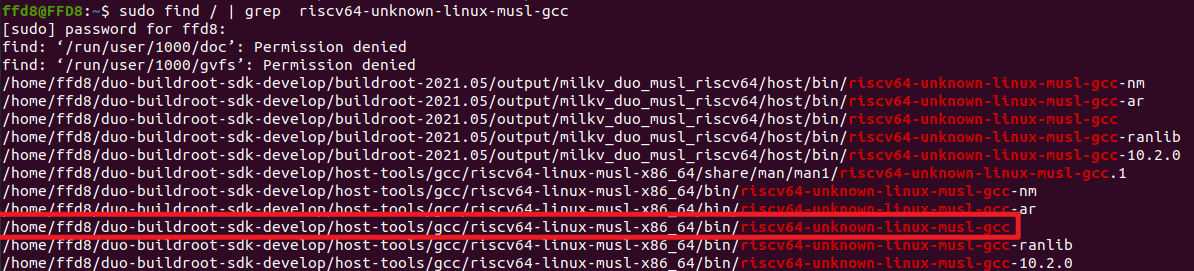
4、编译生成可执行文件
sudo /home/ffd8/duo-buildroot-sdk-develop/host-tools/gcc/riscv64-linux-musl-x86_64/bin/riscv64-unknown-linux-musl-gcc -static -o led led.c

5、传输到Duo开发板
sudo scp ./led root@192.168.42.1:/root/

6、运行程序
程序按照预期打印了30次,同时板载蓝灯同步闪烁
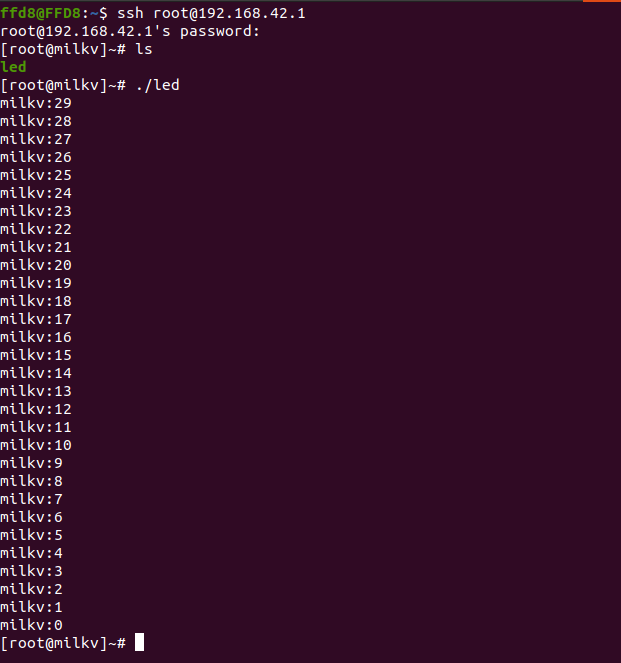
打印
|